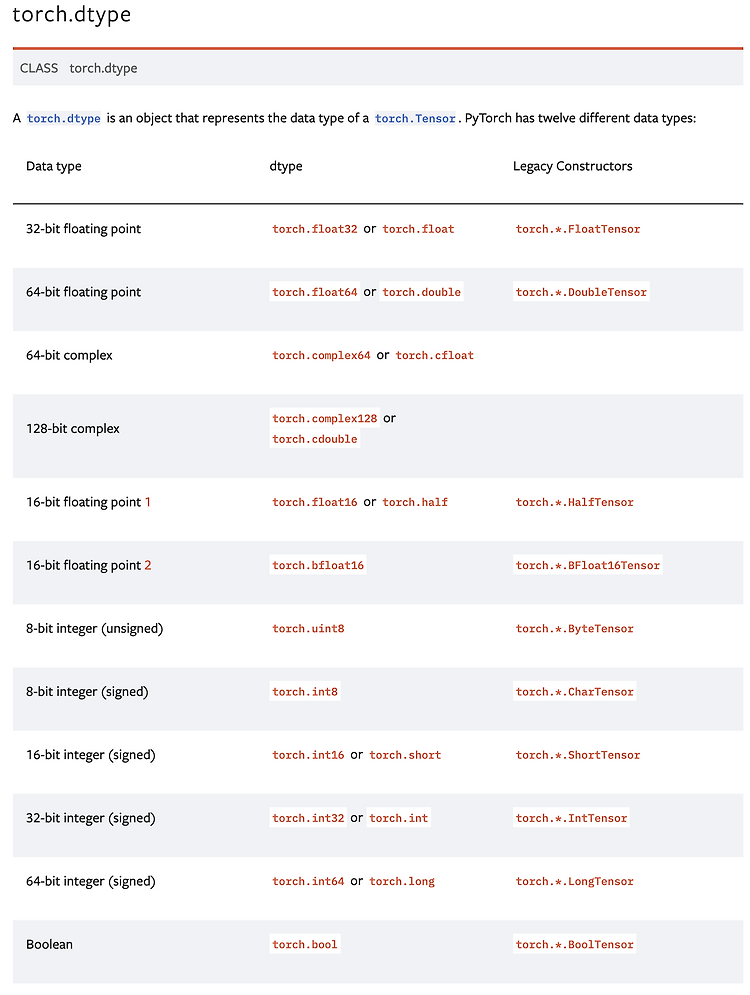
텐서 조작 방법 (Tensor Operations)전공 이론 공부/자연어처리2024. 7. 3. 20:42
Table of Contents
1. torch
import torch # torch import
x= 34.0
print(type(x)) # cpu 레벨에 있는 타입 즉, 파이썬 내의 오브젝트 타입이다.
# output: <class 'float'>
y = torch.tensor(x))
print(type(y)) # tensor로 바꾸는 순간, 파이썬 내부의 오브젝트 타입이 아닌, pytorch 내의 오브젝트 타입이 됨.
# output: <class 'torch.Tensor'>
2. list to Tensor
- list도 텐서로 매핑됨.
- 텐서는 scalar, vector, matrix, n-dimension array를 모두 포함하는 개념이기 때문
x = [1,2,3,4]
y = torch.tensor(x)
print(type(x))
# ouput: <class 'list'>
print(type(y))
# output: <class 'torch.Tensor'>
3. size of tensor
- size는 row, column 순으로 출력된다.
x = [
[1,2,3,4],
[5,6,7,8]
]
print(torch.tensor(x))
# output: tensor([[1,2,3,4],[5,6,7,8]])
print(torch.tensor(x).shape)
# output: tensor.Size([2,4])
print(torch.tensor(x).size())
# output: tensor.Size([2,4])
x = np.array([
[
[
[1,2,3,4],
[5,6,7,8]
]
]
])
print(torch.tensor(x))
# tensor([[[[1,2,3,4],[5,6,7,8]]]], dtype=torch.int32)
print(torch.tensor(x).shape)
# torch.Size([1,1,2,4])
4. Numpy to tensor
import numpy as np
a = 34.3
b = torch.tensor(a)
print(b)
# output: tensor(34.3000)
print(b.dtype)
# output: torch.float32
x = np.array(34.3)
print(type(x))
# output: <class 'numpy.ndarray'>
print(x.dtype)
# output: float64
y = torch.tensor(x)
print(y)
# output: tensor(34.3000, dtype=torch.float64)
5. Type Conversion
1) tensor type
2) tensor type 선언 (create 시 명시)
x = torch.tensor([1,2,3,4], dtype=torch.float)
print(x)
# tensor([1., 2., 3., 4.])
print(x.dtype)
# torch.float32
y = torch.tensor([1,2,3,4], dtype=torch.float16)
3) tensor type conversion
- to() 사용
x = torch.tensor([1,2,3,4], dtype=torch.float)
print(x)
# tensor([1., 2., 3., 4.])
print(x.dtype)
# torch.float32
y = x.to(torch.int)
print(y)
# tensor([1,2,3,4], dtype=torch.int32)
print(y.dtype)
# torch.int32
- type() 사용
x = torch.tensor([1,2,3,4], dtype=torch.float)
print(x)
# tensor([1., 2., 3., 4.])
print(x.dtype)
# torch.float32
y = x.type(torch.int)
print(y)
# tensor([1,2,3,4], dtype=torch.int32)
print(y.dtype)
# torch.int32
6. Tensor Shape
- tensor는 기본적으로 n-dimensional array
- x.size도 결국 텐서
x = torch.tensor(
[
[1,2,3],
[4,5,6]
] )
print(x.shape)
# torch.Size([2,3])
batch_size, dim_1 = x.shape
print(batch_size)
# 2
print(dim_1)
# 3
- 다음과 같이 변환(reshape) 가능
- 모든 컴포넌트가 가지고 있는 정보량이 같다면! (총 컴포넌트 수가 같다면) 변환 가능 - 손실될 정보가 없기 때문에
- 정보량이 같은지는 reshape안에 있는 숫자들 모두 곱했을 때 같은지 확인하면 됨.
print("Original: ", x.shape)
print("Reshaped: ", x.reshape[3,2]).shape)
print("Reshaped: ", x.reshape[1,6]).shape)
print("Reshaped: ", x.reshape[6,1]).shape)
# output
# Original: torch.Size([2,3])
# Reshaped: torch.Size([3,2])
# Reshaped: torch.Size([1,6])
# Reshaped: torch.Size([6,1])
print(x)
# output: tensor([[1,2,3], [4,5,6]])
print(x.reshape[6]))
# output: tensor([1,2,3,4,5,6])
print(x.reshape[1,1,1,1,1,1,1,1,1,6])
# output: tensor([[[[[[[[[[1,2,3,4,5,6]]]]]]]]]])
7. Reshape and View
- 'view'란: 파이토치에서 제공하는 shaping operation. reshape와 거의 비슷하지만 메모리 사용방식이 다름.
- view로 리턴하게 되면 shape는 다르지만 말 그대로 view만 바꿔줌. 데이터 자체의 메모리 구조를 건들이지 않음.
- 데이터를 액세스하게 될 때의 shape만 바꿔주는 것. (data 자체를 바꾸지는 않음!!!)
출처: CNU ISoft Lab (Deep Learning NLP 101)
반응형
'전공 이론 공부 > 자연어처리' 카테고리의 다른 글
임베딩(Embedding)이란? (0) | 2024.07.09 |
---|---|
Transformer v.s. RNN (0) | 2024.07.09 |
BOW (Bag of Words) (0) | 2024.06.30 |
단어 빈도 그래프 (0) | 2024.06.25 |
텍스트 전처리 (Text Preprocessing) (0) | 2024.06.25 |
@쿠몬e :: ˚˛˚ * December☃ 。* 。˛˚
전공 공부 기록 📘
포스팅이 좋았다면 "좋아요❤️" 또는 "구독👍🏻" 해주세요!